Getting to grips with the basics of REST APIs (From REST consumer to API provider 1/3)
Share on socials
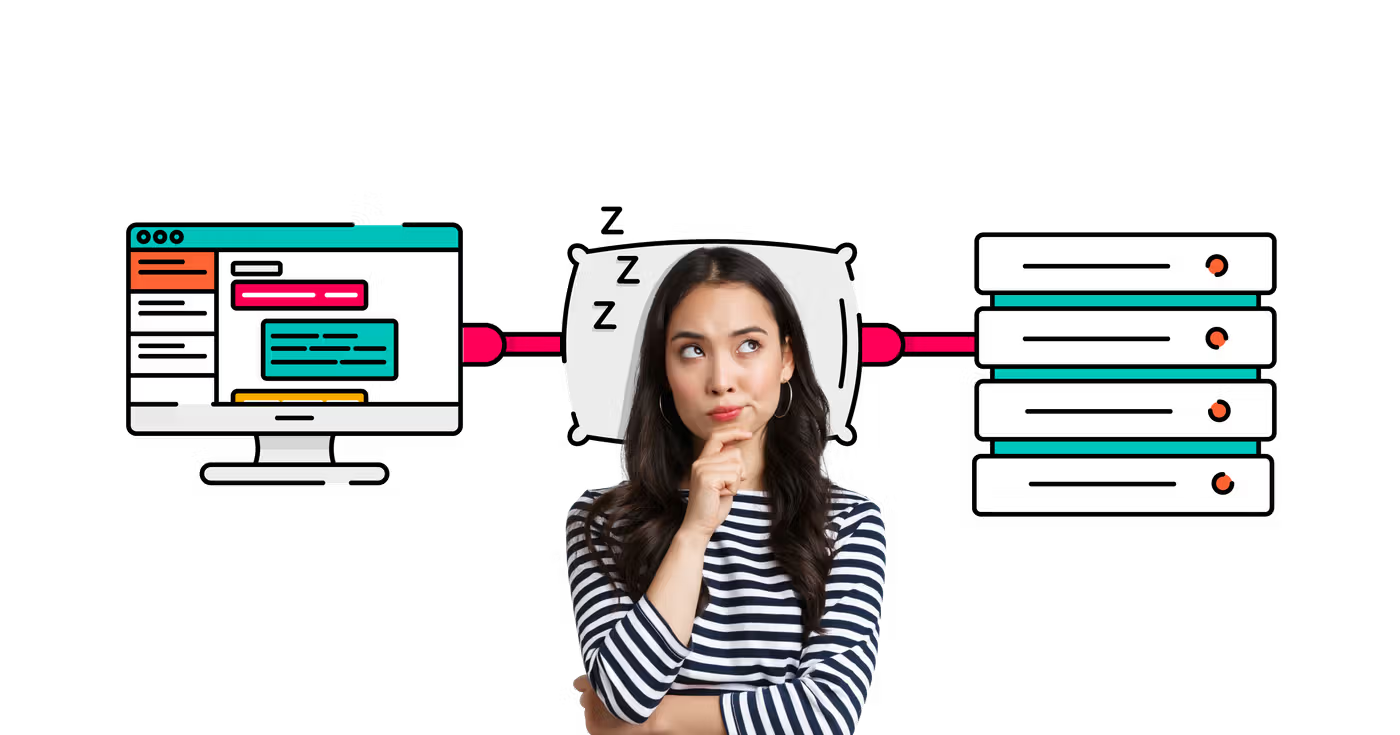
Getting to grips with the basics of REST APIs (From REST consumer to API provider 1/3)
Welcome to the From REST consumer to API provider guest tutorial. In this three-part series, Rafael Pinto Sperafico from The Adaptavist Group partner, Ambientia, will guide you through the world of REST APIs. In this first tutorial, we’ll get you started with the fundamentals of REST APIs. In tutorial two, we’ll introduce you to ScriptRunner Connect, showing how you can simplify and automate your interactions with APIs. Finally, in tutorial three we’ll challenge you to take everything you've learned and apply it to building a managed API.
By the end of this series, you’ll have a strong understanding of REST APIs, experience with tools like ScriptRunner Connect, and the skills to create your own managed APIs. We hope you enjoy the series, where we bring REST APIs to life with ScriptRunner Connect!
Ready to dive in? Let’s begin by exploring the basics of REST APIs!
What you will learn:
1. Introduction to REST APIs using the ReqRes API as an example
2. Core concepts of REST
3. How to make HTTP requests (GET, POST, PUT, PATCH, and DELETE)
4. How to interpret HTTP status codes
What is a REST API?
A REST API (REpresentational State Transfer Application Programming Interface) allows systems to communicate over the web using standard HTTP methods. REST APIs are stateless, meaning each request is processed independently without relying on prior interactions.
Key features of REST APIs:
- Resources: REST treats everything (e.g., users, orders, products) as resources, accessible via URLs.
- HTTP methods: Each method (verb) corresponds to a specific operation on the resource.
- Statelessness: Each API request is self-contained.
- HTTP status codes: Feedback about the success or failure of requests.
HTTP methods and their functions
REST APIs use standard HTTP methods, also known as verbs, to define the type of action to be performed on a resource. Here’s a quick breakdown:
- GET fetches data from the server
- POST creates a new resource
- PUT updates an entire resource
- PATCH updates part of a resource
- DELETE removes a resource
What are HTTP status codes?
HTTP status codes are three-digit numbers included in every API response to indicate the outcome of an HTTP request. They act as a communication bridge between a client (e.g., your browser, application) and a server, informing whether a request was successful, failed, or needs further action.
Status codes are categorised into five groups based on their starting digit:
1xx: Informational
- Indicates that the request was received and is being processed.
- Example:
100 Continue
: The server is ready to receive the rest of the request.
2xx: Success
- Confirms that the request was successfully processed.
- Example:
200 OK
: The request succeeded (e.g., fetching or updating data).201 Created
: A new resource was successfully created.
3xx: Redirection
- Indicates that the client needs to take additional steps (e.g., follow a new URL).
- Example:
301 Moved Permanently
: The resource is now located at a different URL.
4xx: Client errors
- The request is invalid due to an issue on the client's side.
- Example:
400 Bad Request
: The server could not understand the request due to bad syntax.401 Unauthorised
: Authentication is required but not provided or invalid.404 Not Found
: The requested resource does not exist.
5xx: Server errors
- The server encountered an issue while processing the request.
- Example:
500 Internal Server Error
: A generic error indicating the server failed unexpectedly.503 Service Unavailable
: The server is temporarily unable to handle requests.
Why are HTTP status codes important?
- Error diagnosis: Help developers quickly identify and debug issues.
- Actionable feedback: Inform the client about how to proceed (e.g., retry, authenticate).
- Standardisation: Provide a universal language for communication between systems.
When working with APIs, understanding status codes ensures you can interpret responses correctly and handle errors gracefully.
Exploring REST with examples: ReqRes API
GET: Retrieving data
The
GET
method fetches data from the server. With ReqRes API, you can retrieve a paginated list of users or a single user.URL:
https://reqres.in/api/users
ReqRes API supports query parameters for pagination. For example, to fetch users on page 2:
https://reqres.in/api/users?page=2
Example response
{
"page": 2,
"per_page": 6,
"total": 12,
"total_pages": 2,
"data": [
{
"id": 7,
"email": "michael.lawson@reqres.in",
"first_name": "Michael",
"last_name": "Lawson",
"avatar": "https://reqres.in/img/faces/7-image.jpg"
},
...
]
}
Query parameters:
page
: Specifies the page number to fetch.per_page
: Controls the number of items per page (optional).
What is a query parameter?
A query parameter is the part of a URL used to pass additional information to a web server. It allows users or applications to customise their requests by adding key-value pairs at the end of a URL. Query parameters are often used for filtering, searching, sorting, or paginating data in REST APIs.
Structure of query parameters
Query parameters are appended to the URL after a question mark (?), and multiple parameters are separated by an ampersand (&). Each parameter consists of a key and its corresponding value, joined by an equals sign (=).
Syntax:
http://example.com/resource?key1=value1&key2=value2
- Base URL: http://example.com/resource
- Query Parameters:
key1=value1&key2=value2
Example: Pagination with query parameters
When interacting with APIs, query parameters are commonly used to paginate through results. For instance:
URL:
https://reqres.in/api/users?page=2&per_page=5
page=2
: Indicates that you want the second page of results.per_page=5
: Specifies that you want 5 results per page.
Why use query parameters?
Query parameters provide flexibility by allowing users to:
- Filter data: Retrieve resources based on specific conditions (e.g.,
?status=active
). - Sort data: Specify the sorting order (e.g.,
?sort=asc
). - Paginate results: Handle large datasets by dividing them into smaller chunks (e.g.,
?page=3&per_page=10
). - Search resources: Perform searches by keyword (e.g.,
?q=John
).
By using query parameters, APIs can handle a wide range of custom requests without needing additional endpoints.
Key notes
- Web browsers automatically perform GET requests when you type the URL into the address bar.
- Try it by pasting:
https://reqres.in/api/users?page=2
in your browser to see the paginated results.
- Try it by pasting:
- If the API requires authentication, browsers allow you to perform
GET
requests after logging in.
POST: Creating data
To add a new user, use the
POST
method with the user’s details in the request body.URL:
https://reqres.in/api/users
Request Body
{
"name": "Jane Doe",
"job": "Developer"
}
Example with cURL
curl -X POST https://reqres.in/api/users \
-H "Content-Type: application/json" \
-d '{
"name": "Jane Doe",
"job": "Developer"
}'
Example Response
{
"name": "Jane Doe",
"job": "Developer",
"id": "123",
"createdAt": "2024-11-27T10:15:30.123Z"
}
PUT: Replacing data
To completely replace the details of an existing user, use
PUT
. For example, updating user ID 2:URL:
https://reqres.in/api/users/2
Request Body
{
"name": "John Doe",
"job": "Engineer"
}
Example with cURL
curl -X PUT https://reqres.in/api/users/2 \
-H "Content-Type: application/json" \
-d '{
"name": "John Doe",
"job": "Engineer"
}'
Example response
{
"name": "John Doe",
"job": "Engineer",
"updatedAt": "2024-11-27T10:16:45.789Z"
}
PATCH: Partially updating data
For partial updates, use the
PATCH
method. For instance, changing only the job title of user ID 2:URL:
https://reqres.in/api/users/2
Request Body
{
"job": "Senior Engineer"
}
Example with cURL
curl -X PATCH https://reqres.in/api/users/2 \
-H "Content-Type: application/json" \
-d '{
"job": "Senior Engineer"
}'
Example response
{
"name": "John Doe",
"job": "Senior Engineer",
"updatedAt": "2024-11-27T10:17:30.456Z"
}
DELETE: Removing data
To delete a user, send a
DELETE
request. For example, deleting user ID 2:URL:
https://reqres.in/api/users/2
Example with cURL
curl -v -X DELETE https://reqres.in/api/users/2
Example response:
The server will return a
204 No Content
status code, indicating the resource was successfully deleted but no content is returned.Conclusion
REST APIs make it easy to connect anything to everything using a common set of HTTP methods. By understanding the basics of verbs, HTTP status codes, and tools for interaction, you can start building and consuming RESTful services today.
Start practising with the ReqRes API and explore how REST can unlock the full potential of interconnected systems by reading our next chapter: REST APIs made easy with ScriptRunner Connect.
Published on 5 March 2025
Authors
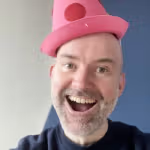
Rafael Pinto Sperafico
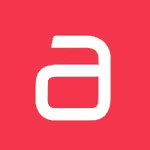
Ambientia
Share this tutorial
Written by
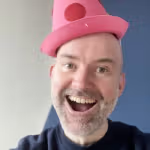
Senior Software Developer
I’m part of the dynamic teams at Ambientia, working at the intersection of innovation and technology. With a passion for APIs, automation, and developer enablement, I’m excited to guide you through the fundamentals of REST APIs and how they can transform the way applications communicate and integrate by using ScriptRunner Connect.
Connect with Rafael on LinkedIn.
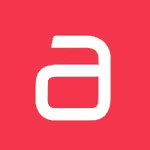
Ambientia is a Finnish technology consultancy, partners with organizations to create smart digital solutions. With a focus on collaboration, automation, and user-centric design, Ambientia brings cutting-edge technologies to life, helping companies across various industries to innovate and grow sustainably.
Related content
Discover more like this

REST APIs made easy with ScriptRunner (From REST consumer to API provider 2/3)
Welcome to the From REST consumer to API provider guest tutorial. In this three-part series, Rafael Pinto Sperafico from The Adaptavist Group partner, Ambientia, will guide you through the world of REST APIs. In this tutorial we’ll explore how to automate REST API calls using ScriptRunner Connect.
by Rafael Pinto Sperafico
on 5 Mar 2025
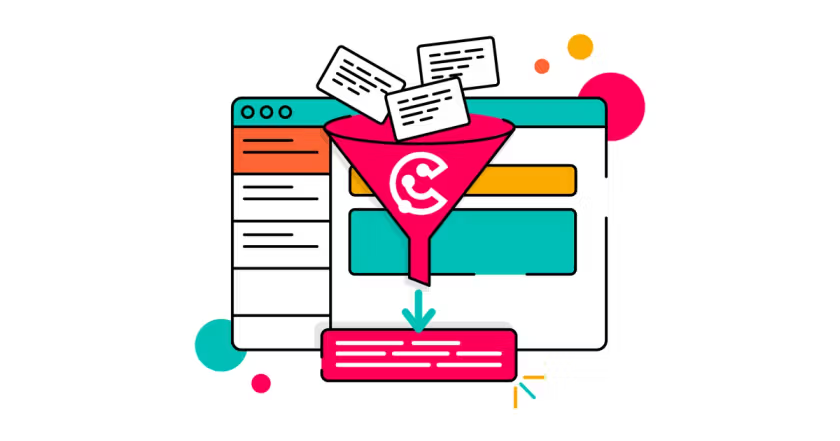
Building a managed API (From REST consumer to API provider 3/3)
Welcome to the From REST consumer to API provider guest tutorial. In this three-part series, Rafael Pinto Sperafico from The Adaptavist Group partner, Ambientia, will guide you through the world of REST APIs. In this tutorial, we transform consumer-oriented scripts into a reusable, managed API, we'll build a TypeScript-based package that encapsulates the same operations, making the logic portable and maintainable. Additionally, we’ll demonstrate how to publish the managed API to npm and integrate it into ScriptRunner Connect.
by Rafael Pinto Sperafico
on 5 Mar 2025