REST APIs made easy with ScriptRunner (From REST consumer to API provider 2/3)
Share on socials
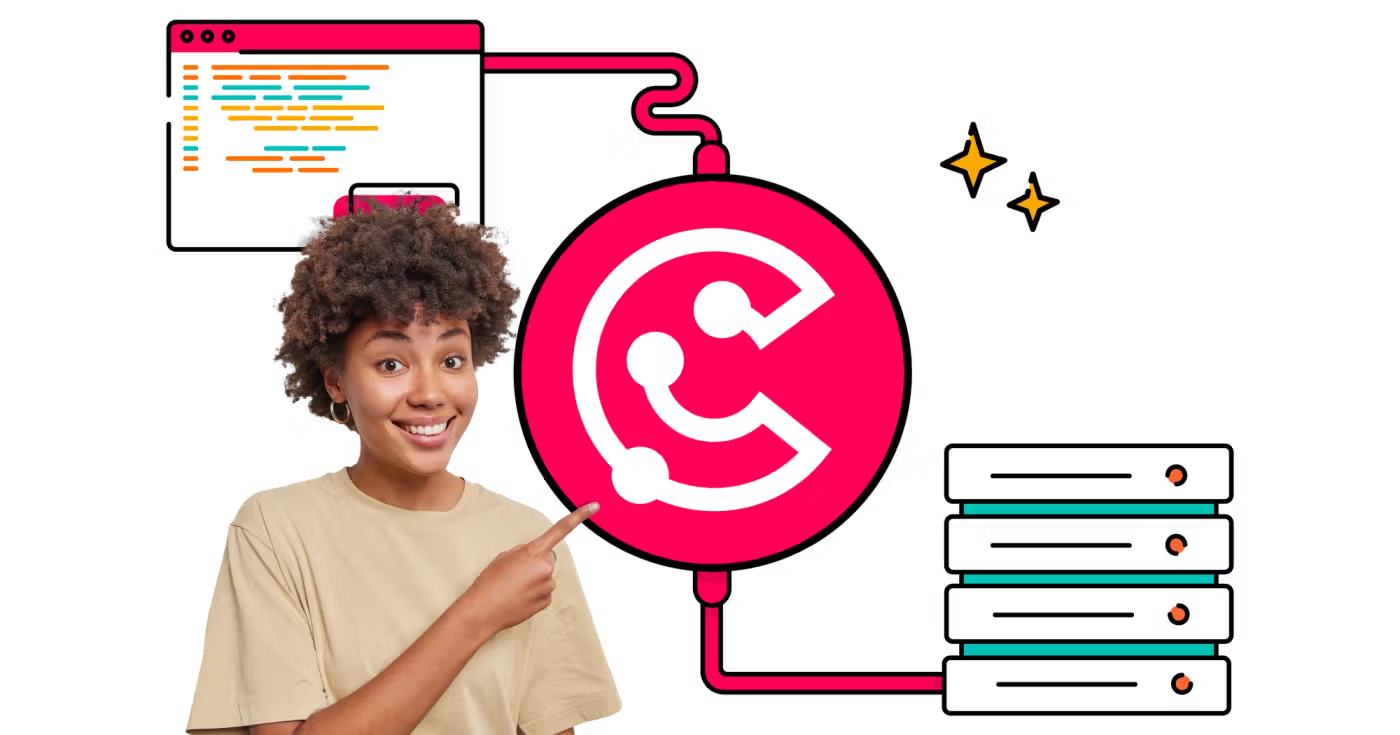
REST APIs made easy with ScriptRunner (From REST consumer to API provider 2/3)
Welcome to the From REST consumer to API provider guest tutorial. In this three-part series, Rafael Pinto Sperafico from The Adaptavist Group partner, Ambientia, will guide you through the world of REST APIs. In the previous post, Getting to grips with the basics of REST APIs, we got you started with the fundamentals.
Now, we’ll explore how to automate REST API calls using ScriptRunner Connect. We’ll use the ReqResAPI as our example, demonstrating how to set up GET, POST, PUT, PATCH, and DELETE requests in ScriptRunner Connect. By the end of this guide, you’ll know how to configure ScriptRunner Connect to interact with APIs, create and run scripts, and automate them using scheduled triggers.
What you will learn:
1. How to set up your ScriptRunner Connect account
2. How to set up a Generic Connector
3. How to set up GET, POST, PUT, PATCH, and DELETE requests in ScriptRunner Connect
4. How to automate scripts with scheduled triggers
Step 1: Creating your ScriptRunner Connect account
To start using ScriptRunner Connect, you’ll need an account. Here’s how to create one:
- Navigate to the ScriptRunner Connect sign-in page.
- Click Sign up and provide your email address and a password to create an account.
- Verify your email by clicking the confirmation link sent to your inbox.
- Once verified, log in to your new ScriptRunner Connect account.
Reminder: check your ScriptRunner Connect version!
Please note, this tutorial was published on 4 March 2025 and screenshots/instructions may appear differently in future versions.
Step 2: Creating a team
Teams in ScriptRunner Connect help you collaborate and manage your automation projects.
- After logging in, click on Teams in the main menu.
- Click + New team and provide a name for your team.
- When prompted to choose a plan, select the Free tier option. This tier is perfect for exploring and testing the platform.
Step 3: Creating a workspace
Workspaces are where you build and manage your scripts, connectors, and configurations.
- Go to the Workspaces tab.
- Click + Create new and give it a name, such as "ReqRes API Workspace"
- Select your newly created team to associate the workspace with your team.
Step 4: Setting up a Generic Connector
To make requests to the ReqRes API, we need to create a Generic Connector.
- Navigate to the Connectors tab in your workspace.
- Click Create connector and select Generic.
- Configure the connector:
- Name: "ReqRes API Connector"
- Base URL: https://reqres.in/api/
- Leave other fields as default.
- Click Save connector to finalise the setup.
Your Generic Connector is now ready to make HTTP requests to ReqRes API!
Step 5: Creating a script
Now we’ll create a script to make
GET
, POST
, PUT
, PATCH
, and DELETE
requests to the ReqRes API.- From your Workspace, navigate to the Scripts section.
- Click + to create a new script, give it a name (e.g., "reqresScript").
With the Generic Connector configured, importing it to your project cannot be easier:
- Go to the Workspaces tab
- Select the workspace created, e.g "ReqRes API Workspace"
- Under API Connections, click on + to create a new API connection
- From Uses Connector, select "ReqRes API Connector"
Remember to save your script when changes to the code are made.
Import API connection
To make use of our connector in our script, we must import it:
- Open your Script "reqresScript"
- On the top right-hand side of the screen, select the down arrow to import API connection.
- Select the API Connection: ReqRes API Connector
- Give an Import name, e.g ReqResClient
You can identify your connector in your script by the import:
reqresScript
import ReqResClient from './api/generic';
export default async function (event: any, context: Context): Promise<void> {
console.log('Script triggered', event, context);
}
Remember to save your script when changes to the code are made.
GET request: Fetch users
In this script, the query parameter
page=2
is hardcoded to fetch the second page of users from the ReqRes API. Query parameters like page
allow us to paginate through results, retrieving different sets of data based on the value provided.reqresScript
import ReqresInClient from './api/generic';
export default async function (event: any, context: Context): Promise<void> {
console.log('Script triggered', event, context);
const users = await getUsers(2);
// Print out the users
console.log('Users', users);
}
const getUsers = async (pageNumber: number) => {
// Fetch users
const response = await ReqresInClient.fetch(`/users?page=${pageNumber}`);
// Check if the response is OK
if (!response.ok) {
// If it's not, then throw an error
throw Error(`Unexpected response code: ${response.status} - ${await response.text()}`);
}
console.log(`HTTP Status Code: ${response.status}`);
// If it's OK, then proceed by getting the JSON object from the body that represents the users
return await response.json();
}
To fetch all pages dynamically, we would need to rewrite this script to loop through all available pages by inspecting the REST API response (which contains the total number of pages). However, we’ll dive into how to do this in the next post, Building a managed API, where we’ll enhance this script to fetch all pages and explore how to package this logic into a reusable and managed API for broader applications.
POST request: Create a user
This script demonstrates how to create a user via the ReqRes API using the
POST
method.reqresScript
import ReqresInClient from './api/generic';
export default async function (event: any, context: Context): Promise<void> {
console.log('Script triggered', event, context);
// const users = await getUsers(2);
//
// Print out the users
// console.log('Users', users);
const newUser = await createUser();
// Print out the user created
console.log('New User', newUser);
}
const getUsers = async (pageNumber: number) => { ... }
const createUser = async () => {
// Create User
const response = await ReqresInClient.fetch('/users', {
method: 'POST',
headers: {
'Content-type': 'application/json'
},
body: JSON.stringify({
name: 'John Doe',
job: 'Software Engineer',
})
});
// Check if the response is OK
if (!response.ok) {
// If it's not, then throw an error
throw Error(`Unexpected response code: ${response.status} - ${await response.text()}`);
}
console.log(`HTTP Status Code: ${response.status}`);
// If it's OK, then proceed by getting the JSON object from the body that represents the created user
return await response.json();
}
However, there are two important considerations to note:
1. Status code
When creating a resource in most real-world APIs, you would expect a status code of
201 Created
, which indicates that a new resource has been successfully created on the server. In this case, the ReqRes API returns a status code of 200 OK
. This is because ReqRes is a simulation API meant for testing purposes only. It doesn’t actually create resources but simulates the behaviour for educational and development use.2. Static payload
In this example, the payload —
name
and job
— is hardcoded. While this works for this demonstration, dynamic payloads allow more flexible and practical solutions. We will address this in the next post, where we’ll enhance the script to accept dynamic input for creating users.PUT request: Update a user
This script demonstrates how to update a user via the ReqRes API using the
PUT
method.reqresScript
import ReqresInClient from './api/generic';
export default async function (event: any, context: Context): Promise<void> {
console.log('Script triggered', event, context);
// const users = await getUsers(2);
//
// // Print out users
// console.log('Users', users);
// const newUser = await createUser();
//
// // Print out the created user
// console.log('New User', newUser);
const updatedUser = await updateUser(2);
// Print out the updated user
console.log('Updated User', updatedUser);
}
const getUsers = async (pageNumber: number) => { ... }
const createUser = async () => { ... }
const updateUser = async (userId: number) => {
// Update User
const response = await ReqresInClient.fetch(`/users/${userId}`, {
method: 'PUT',
headers: {
'Content-type': 'application/json'
},
body: JSON.stringify({
name: 'John Doe',
job: 'Senior Developer',
})
});
// Check if the response is OK
if (!response.ok) {
// If it's not, then throw an error
throw Error(`Unexpected response code: ${response.status} - ${await response.text()}`);
}
console.log(`HTTP Status Code: ${response.status}`);
// If it's OK, then proceed by getting the JSON object from the body that represents the updated user
return await response.json();
}
In this example, the path parameter —
2
— and payload — name
and job
— are hardcoded. While this works for this demonstration, dynamic path parameter and payloads allow more flexible and practical solutions. We will address this in the next post, Building a managed API, where we’ll enhance the script to accept dynamic input for updating users.PATCH request: Partially update a user
This script demonstrates how to update a user via the ReqRes API using the
PATCH
method.reqresScript
import ReqresInClient from './api/generic';
export default async function (event: any, context: Context): Promise<void> {
console.log('Script triggered', event, context);
// const users = await getUsers(2);
//
// // Print out users
// console.log('Users', users);
// const newUser = await createUser();
//
// // Print out the created user
// console.log('New User', newUser);
// const updatedUser = await updateUser(2);
//
// // Print out the updated user
// console.log('Updated User', updatedUser);
const patchedUser = await patchUser(2);
// Print out the patched user
console.log('Patched User', patchedUser);
}
const getUsers = async (pageNumber: number) => { ... }
const createUser = async () => { ... }
const updateUser = async (userId: number) => { ... }
const patchUser = async (userId: number) => {
// Patch User
const response = await ReqresInClient.fetch(`/users/${userId}`, {
method: 'PATCH',
headers: {
'Content-type': 'application/json'
},
body: JSON.stringify({
job: 'Senior Developer',
})
});
// Check if the response is OK
if (!response.ok) {
// If it's not, then throw an error
throw Error(`Unexpected response code: ${response.status} - ${await response.text()}`);
}
console.log(`HTTP Status Code: ${response.status}`);
// If it's OK, then proceed by getting the JSON object from the body that represents the patched user
return await response.json();
}
In this example, the path parameter —
2
— and payload — job
— are hardcoded. While this works for this demonstration, dynamic path parameter and payloads allow more flexible and practical solutions. We will address this in the next post, Building a managed API, where we’ll enhance the script to accept dynamic input for patching users.DELETE request: Remove a user
This script demonstrates how to update a user via the ReqRes API using the
DELETE
method.reqresScript
import ReqresInClient from './api/generic';
export default async function (event: any, context: Context): Promise<void> {
console.log('Script triggered', event, context);
// const users = await getUsers(2);
//
// // Print out users
// console.log('Users', users);
// const newUser = await createUser();
//
// // Print out the created user
// console.log('New User', newUser);
// const updatedUser = await updateUser(2);
//
// // Print out the updated user
// console.log('Updated User', updatedUser);
// const patchedUser = await patchUser(2);
//
// // Print out the patched user
// console.log('Patched User', patchedUser);
await deleteUser(2);
}
const getUsers = async (pageNumber: number) => { ... }
const createUser = async () => { ... }
const updateUser = async (userId: number) => { ... }
const patchUser = async (userId: number) => { ... }
const deleteUser = async (userId: number) => {
// Delete User
const response = await ReqresInClient.fetch(`/users/${userId}`, {
method: 'DELETE'
});
// Check if the response is OK
if (!response.ok) {
// If it's not, then throw an error
throw Error(`Unexpected response code: ${response.status} - ${await response.text()}`);
}
console.log(`HTTP Status Code: ${response.status}`);
return true;
}
There are two important considerations to note:
1. Expected status code
When a
DELETE
request is successful in most real-world APIs, the response should return a status code of 204 No Content
to indicate the resource has been deleted successfully, and there is no additional content in the response body. The script above checks for this status code and throws an error if the response code is anything else.2. Static path parameter
In this example, the
DELETE
request is hardcoded to remove the user with ID 2
. While sufficient for this demonstration, using a dynamic path parameter would make the script more flexible, allowing the deletion of any user by specifying their ID programmatically.We’ll tackle this in the next post, Building a managed API, where we’ll rewrite this script to accept dynamic input, making it more reusable and adaptable.
Step 6: Running the script
- In the Scripts tab, locate the script you created.
- Click the Run script button.
- Check the logs to view the output of your script, such as API responses or errors.
You can manually test each HTTP verb by modifying and re-running the script.
Step 7: Automating scripts with scheduled triggers
To automate your scripts, set up a scheduled trigger:
- From your Workspace, go to the Scheduled triggers section.
- Click + to create a new scheduled trigger.
- Configure the trigger:
- Generate CRON expression: Set the desired schedule (e.g., every day at 8:00 AM).
- Run script: Select the script you created.
- Save the trigger.
Your script will now run automatically according to the schedule you defined.
Conclusion
By following these steps, you’ve set up ScriptRunner Connect to interact with the ReqRes API, making
GET
, POST
, PUT
, PATCH
, and DELETE
requests. You’ve also automated the script with a scheduled trigger, making it easier to perform repetitive tasks without manual intervention.ScriptRunner Connect’s flexibility and ease of use make it a powerful tool for integrating with REST APIs and automating workflows.
In the last tutorial in the series, Building a managed API, you’ll learn how to move from REST Consumer to API Provider!
Published on 5 March 2025
Authors
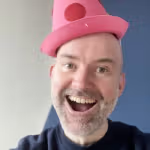
Rafael Pinto Sperafico
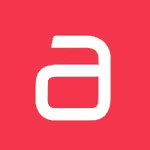
Ambientia
Share this tutorial
Written by
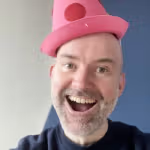
Senior Software Developer
I’m part of the dynamic teams at Ambientia, working at the intersection of innovation and technology. With a passion for APIs, automation, and developer enablement, I’m excited to guide you through the fundamentals of REST APIs and how they can transform the way applications communicate and integrate by using ScriptRunner Connect.
Connect with Rafael on LinkedIn.
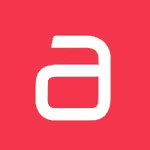
Ambientia is a Finnish technology consultancy, partners with organizations to create smart digital solutions. With a focus on collaboration, automation, and user-centric design, Ambientia brings cutting-edge technologies to life, helping companies across various industries to innovate and grow sustainably.
Related content
Discover more like this
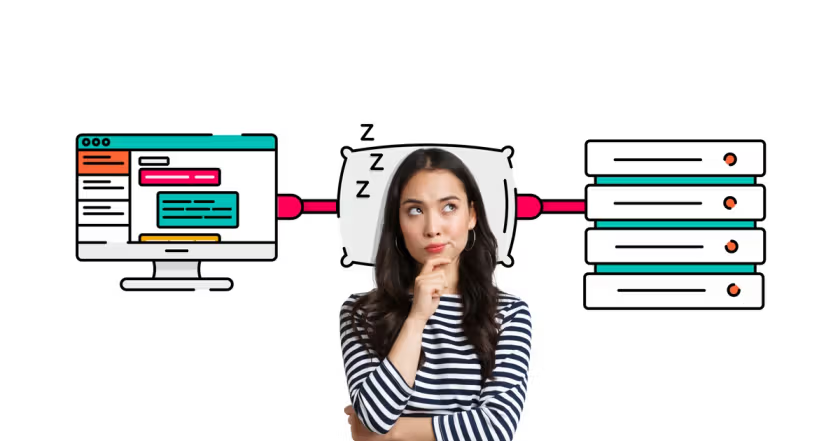
Getting to grips with the basics of REST APIs (From REST consumer to API provider 1/3)
Welcome to the From REST consumer to API provider guest tutorial. In this three-part series, Rafael Pinto Sperafico from The Adaptavist Group partner, Ambientia, will guide you through the world of REST APIs.
by Rafael Pinto Sperafico
on 5 Mar 2025
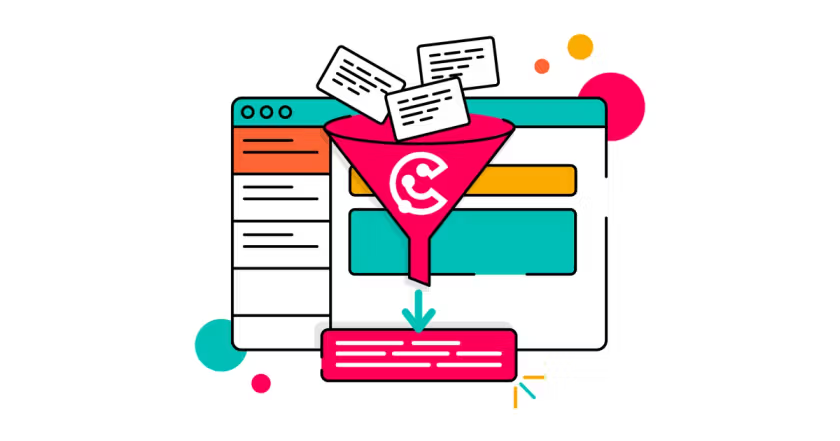
Building a managed API (From REST consumer to API provider 3/3)
Welcome to the From REST consumer to API provider guest tutorial. In this three-part series, Rafael Pinto Sperafico from The Adaptavist Group partner, Ambientia, will guide you through the world of REST APIs. In this tutorial, we transform consumer-oriented scripts into a reusable, managed API, we'll build a TypeScript-based package that encapsulates the same operations, making the logic portable and maintainable. Additionally, we’ll demonstrate how to publish the managed API to npm and integrate it into ScriptRunner Connect.
by Rafael Pinto Sperafico
on 5 Mar 2025